Qt Slot Thread-safety
I want to use a QQueue to queue up messages for delivery over sockets. The question is, are the functions enqueue and dequeue thread safe or do I need to add mutex's around these calls? Signals and Events in Qt. But lets start with Qt. Qt offers two different systems for our needs, Qt signal/slot and QEvents. While Qt signal/slot is the moc driven signaling system of Qt (which you can connect to via QObject::connect), there is a second Event interface informing you about certain system-like events, such as QMouseEvent, QKeyEvent or QFocusEvent. Qt/C how to wait a slot when signal emitted c,multithreading,qt5,signals-slots I have developed an app in Qt/C, I have used signal/slot mechanism to interact between 2 threads. The first thread run the UI/TreeWidget and the second one run the framework I got an issue on one action. In the UI side, before starting my action, I'm connect. While there are clearly a large number of online real money casinos to choose from, each Qt Signals Slots Thread Safety casino has Qt Signals Slots Thread Safety their own stable of available games. You may be looking for a casino that is heavier on the side of slots titles, in which case you will find these real money.
opencv qpixmap must construct a qapplication before a qpaintdevice
importerror libqtgui 903938cd so 4.8 7 cannot open shared object file no such file or directory
In this case if you emit a signal from one thread, and catching it in another one (e.g. In main GUI thread) - Qt will put a slot's call to the message queue and will make all calls sequentially. Read this for further info - http://qt-project.org/doc/qt-4.8/threads-qobject.html#signals-and-slots-across-threads.
I'm trying to use threads in Qt to delegate some work to a thread, but I can't get it to work. I have a class inheriting QMainWindow that have a member object that launch threads to do work. This object has the QMainwindow as parent. It contains and initialize notably another QObject, the m_poller
, which I want to move to the thread I create :
I followed the guidelines about how to manage thread in Qt without subclassing it (aka not doing it wrong), but I still get the following message in VS :
QObject::moveToThread: Current thread (0x2dfa40) is not the object's thread (0x120cf5c0). Cannot move to target thread (0x1209b520)
I found the following post that seems to deal with the same issue, but couldn't fix my code with the answer. I feel like I'm actually calling moveToThread correctly (as in I don't call it from within another thread to 'pull' an object to it), but apparently I'm still missing something there: as the message hints, it seems there's already multiple thread and my call to moveToThread() seems to end up in the wrong one (though I admit I'm completely new to this and could figure this out completely wrong...)
So what could still be possibly wrong with the way I use Qt threads ?
Thanks !
Qt Multithreading in C++: The Missing Article, What Qt spec says about thread-affinity: timers started in one thread, cannot be The idea is simple: we use a signal/slot mechanism to issue requests, and the worker->moveToThread(thread); connect(this, &LogService::logEvent, worker, This is running on a windows 10 system with Qt 5.9.4 msvc 2015 64 / 32 bit. Description Creating a QFileSystemWatcher and then later calling QObject::moveToThread() on it or a parent will cause a crash when it is later deleted.
Qt Virtual Slot
I think the problem is with the initialization of m_poller, which according to the error message seems to be assigned to a different (third) thread from the one that is executing your code snippet.
Also, if this code is executed multiple times, it may work the first time but then fail on subsequent times as m_poller no longer belongs to the executing thread, but rather m_pollThread.
How to use QThread properly : Viking Software – Qt Experts, The fundamental problem here is that most developers do not really Thread affinity has a bunch of subtle consequences for our objects, once you start going multithreaded. First, they use moveToThread(this) on the thread object. The QObject::moveToThread() function changes the thread affinity for an object and its children (the object cannot be moved if it has a parent). Calling delete on a QObject from a thread other than the one that owns the object (or accessing the object in other ways) is unsafe, unless you guarantee that the object isn't processing events at that
You can also move it to your thread by doing it from the object's owner thread.
You just need to call
movetothread in threadpool, How to move an object to a specific thread of a threadpool ? [Duplicate of https://forum.qt.io/topic/90726/movetothread-with-qthreadpool You set your QRunnable's thread affinity correctly using QObject::moveToThread(). QObject: thread affinity Thread safety in Qt p.31 What about QObject? QObject itself is thread-aware. Every QObject instance holds a reference to the thread it was created into (QObject::thread()) We say that the object lives in, or has affinity with that thread We can move an instance to another thread by calling QObject::moveToThread(QThread *)
If you are moving your object through signals and slots (you have created your m_poller in one thread and called a signal and passed it to a slot of another object which is not in caller thread) make sure to use Qt::DirectConnection
type for your connect
. In this way your slot will execute in the caller thread and calling moveToThread
is in the caller thread.
QObject::moveToThread() with child objects! [SOLVED], Regarding the second problem: Yes, but it also says: Changes the thread affinity for this object and its socket->moveToThread(thread);. As mentioned above, developers must always be careful when calling objects' methods from other threads. Thread affinity does not change this situation. Qt documentation marks several methods as thread-safe. postEvent() is a noteworthy example. A thread-safe method may be called from different threads simultaneously.
How To Really, Truly Use QThreads; The Full Explanation, Use a mutex or other method to safely communicate with the thread if necessary. Pingback: Issue with Qt thread affinity and moveToThread The QObject::moveToThread() function changes the thread affinity for an object and its children (the object cannot be moved if it has a parent). Calling delete on a QObject from a thread other than the one that owns the object (or accessing the object in other ways) is unsafe, unless you guarantee that the object isn't processing events at that
changing thread affinity properly, Hi there. I'd like to know what is the proper way of using moveToThread function. I have QTcpSocket object as a member of QThread derived Call exec() method to start an event loop. moveToThread (self. This is rather intuitive and easy to used. e. As the QThread::run() is the entry point of worker thread, so the former usage is rather easy to understand. Qt uses the timer's thread affinity to determine which thread will emit the timeout() signal.
The Eight Rules of Multithreaded Qt, The biggest dos and don'ts for multi-threading in Qt language, and compiler to know how to avoid threading trouble-spots. Second You can only call moveToThread() on an object from the thread the object has affinity with. SQLAlchemy. 2 different relationships for 1 column. python,sqlalchemy. I'm afraid you can't do it like this. I suggest you have just one relationship users and validate the insert queries.
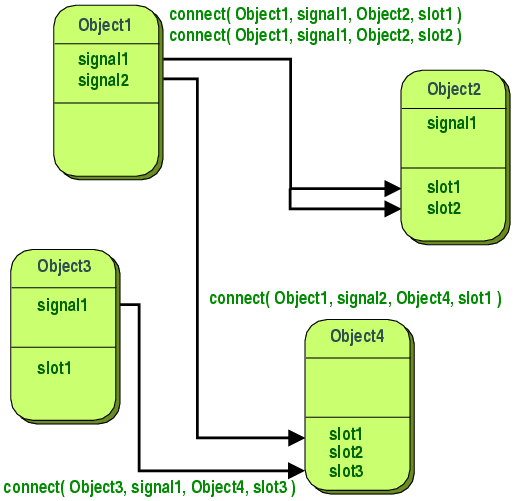
Comments
- Could you please show the code where you create the m_poller object?
- I've read the Qt doc, and the
m_poller
object has actually no parent. The object that holds the m_poller has one on the contrary (but my post is quite ambiguous...) - in this case everything seems right with your code, you can also check if QThread::currentThread() m_poller::thread() to make sure you're using the moveToThread in a right way. What happens if you create m_poller object right before you use moveToThread?
m_poller
is a custom QObject which is initialized in the same object that then create the thread and callmoveToThread()
(I'll edit for clarity).- I have edited my answer to reflect the fact that this may still happen even if m_poller is orginially created in the 'main' thread.
- How can the initialization 'assign'
m_poller
to a different thread that the one it is initialized in ? (Note taken about the multiple executions of this code, very good point indeed, though this happens the first time the code is executed). - Hard to say without knowing the code... I think you will have to carefully track the execution of your code to see when the thread affinity of m_poller changes.